Thread
A Thread represents a group of interactions between a user and an AI app. It can be, for example, a conversation between a human and one or several assistants, or an agent generating a document piece by piece from a user input.
A Thread is composed of Steps. A Step can be a message, or an intermediate AI step like an LLM call.
You can interact with an example Thread in the platform here. This Thread is an example of a user interacting with the chatbot on the Chainlit documentation website. It is a Retrieval Augmented Generation (RAG) application, where first a tool is called to retrieve relevant documentation and cookbook pages from the website. The GPT-4o model is then called to generate a response, using the retrieved context.
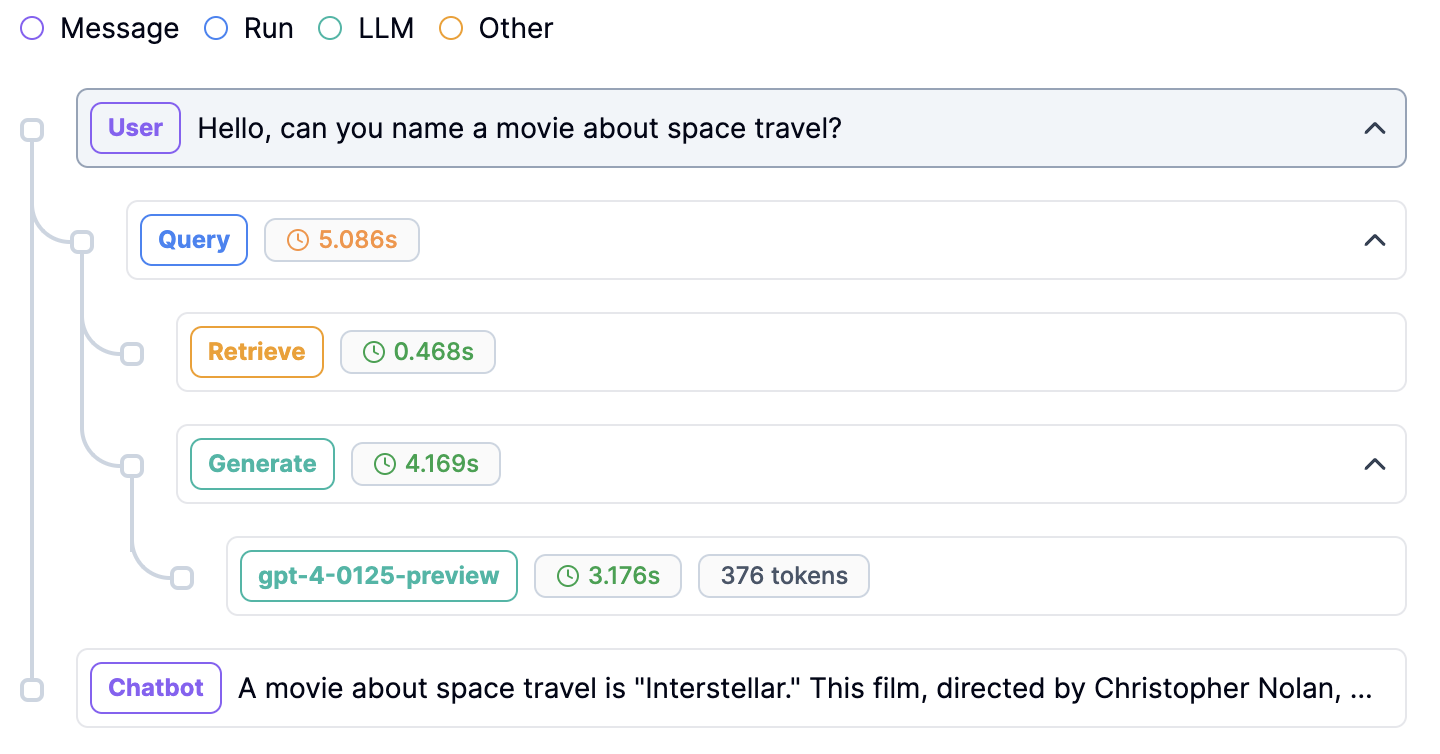
An example thread on the platform.
Log a Thread
There are multiple ways to log Threads in your application. If you are using Python, the simplest way to log a Thread is to use the @literal_client.thread
decorator before a function.
A more flexible option is to use the Python context manager with
statement. More information about ways of logging Threads in Python applications, visit this page.
In TypeScript, you can wrap your applicative code in a Thread using literalClient.thread().wrap(...)
.
List Threads
You can get all the threads from Literal AI to your program:
Here’s an example to use this function with pagination and filters:
Visualize Threads on Literal AI
Navigate to the Threads
page on the platform to see Threads. There, you can click on single Threads (conversations). Click here for an interactive example.
On the left (1), you can see the conversation and the steps that the application has taken in order to produce an answer. Different kind of steps have different colors. You can click on each step to view more details on the right side of the screen. Here, you can view Metadata (2) and add Scores (3).
On the top (4), you can add tags to the Thread. You can also add Tags to a single step in this Thread, on the right (5).
Finally, you can add a Step to a Dataset (6), for example for evaluation purposes.
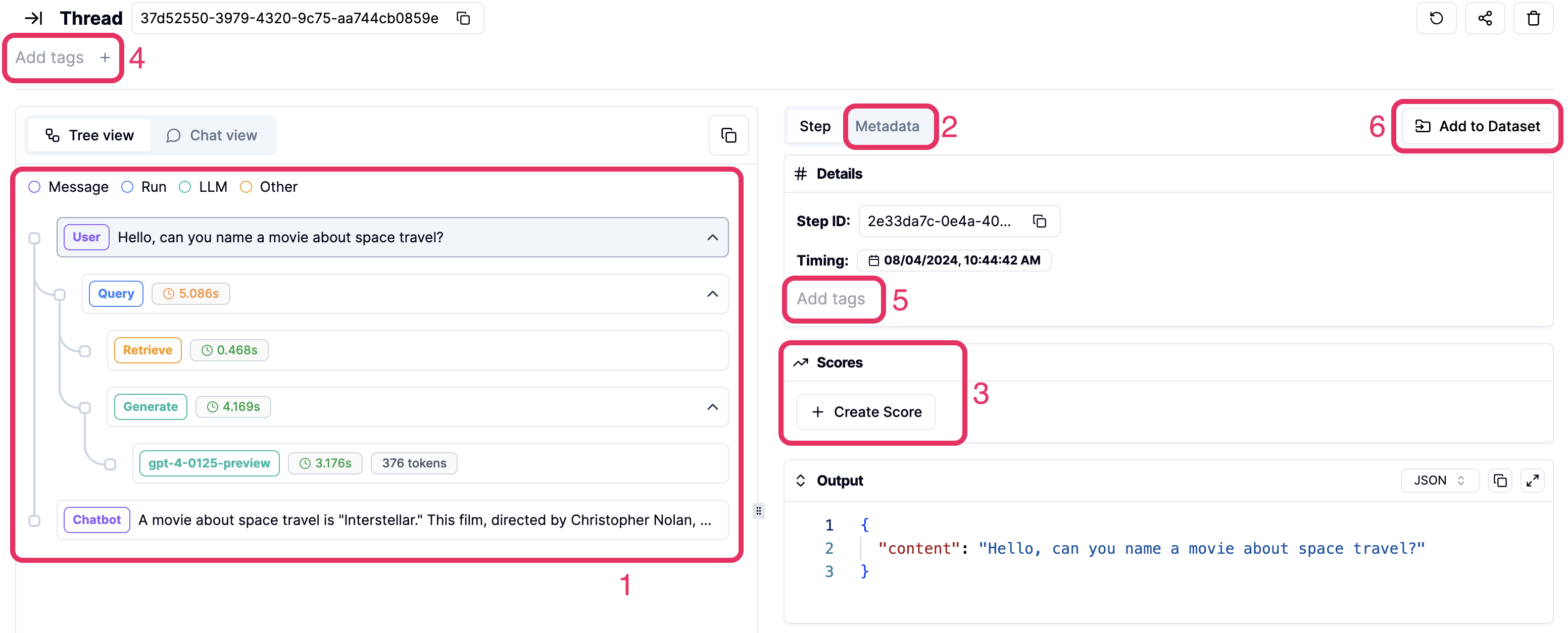
Visualizing a Thread
Tags
You can add Tags to Threads, Steps and Generations. Visit this page for information on how to use Tags.
Was this page helpful?